Setup
Script
Add a scripting block with the following script:
// Get an API key from OMDB here: http://www.omdbapi.com/apikey.aspx
const OMDB_API_KEY = 'YOUR_API_KEY';
// Shows all the button groups together and waits for a button click
// Returns the first button that was clicked
async function chooseFromButtonGroups(label, buttonGroups) {
output.markdown(`## ${label}`);
return await Promise.race(buttonGroups.map(([label, options]) =>
input.buttonsAsync(label, options)
));
}
async function addTitle(title) {
const info = await fetch(`https://www.omdbapi.com/?t=${title}&apikey=${OMDB_API_KEY}`);
const { Title, Runtime, Plot, Poster } = await info.json();
output.markdown(`

# ${Title}
${Plot}
Runtime: \`${Runtime}\`
`);
const table = base.getTable('To watch');
await table.createRecordAsync({
'Name': Title,
'Notes': Plot,
'Attachments': [{
url: Poster,
filename: `${Title}.png`,
}]
});
}
const title = await chooseFromButtonGroups('What would you like to watch next?', [
['TV Comedies', ['The Office', 'Parks and Recreation', 'Frasier']],
['British TV Shows', ['Great British Baking Show', 'Taskmaster']],
['Continue Watching', ['Jeopardy!', 'The West Wing']],
]);
output.clear();
await addTitle(title);
Output
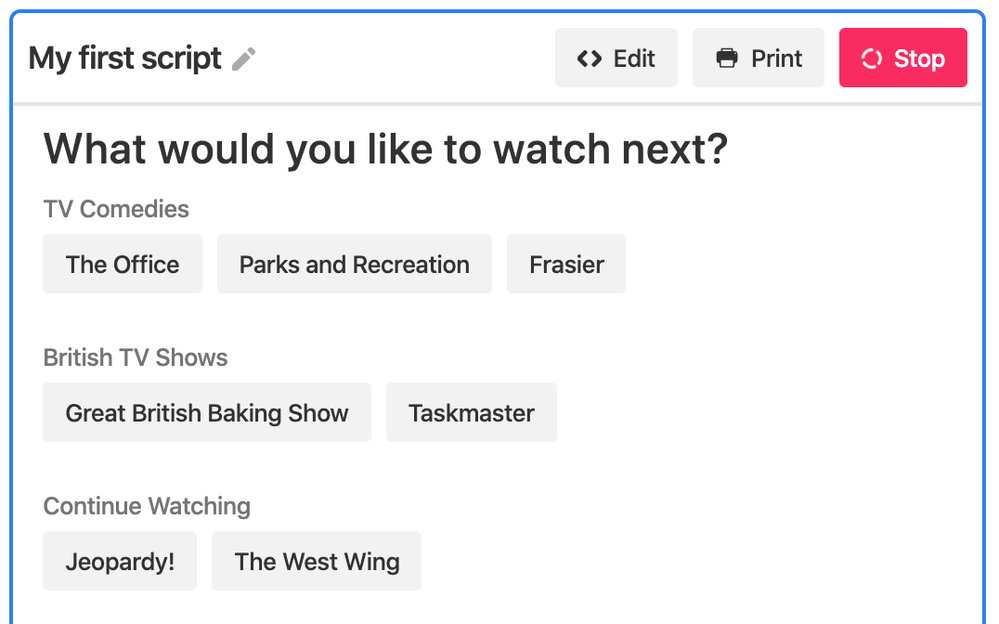
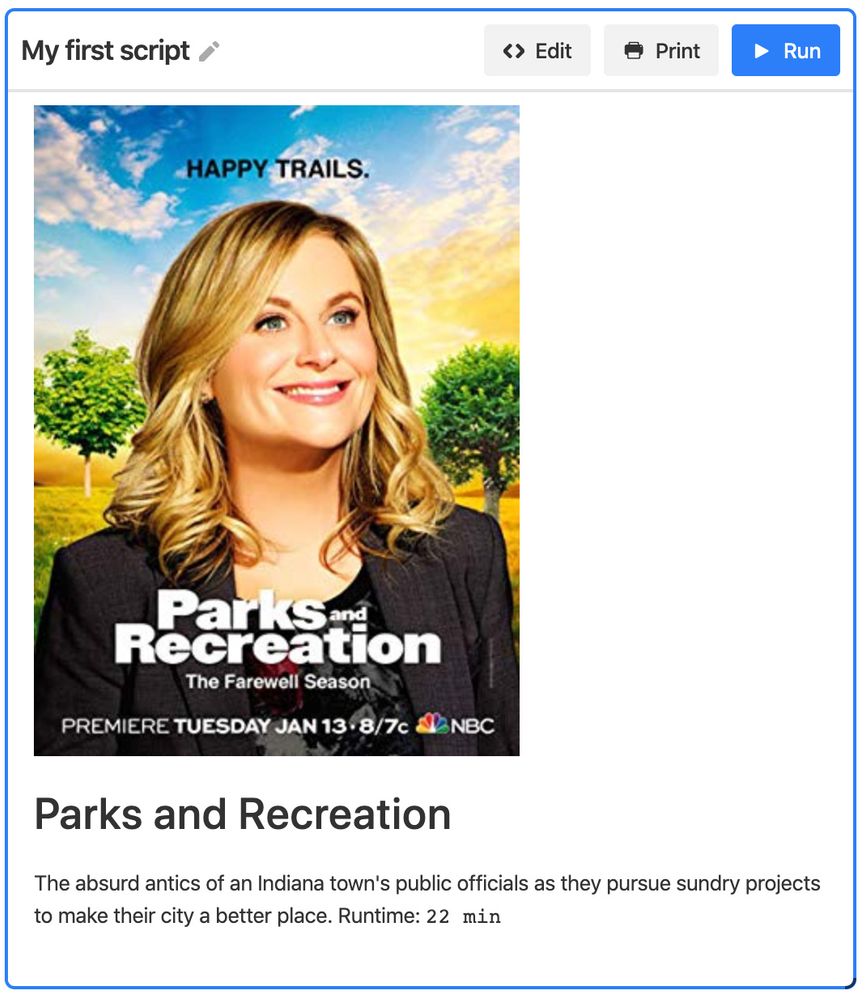
You should see rows of buttons with TV shows in different categories. Clicking on one should fetch the description and poster from OMDB and insert a new record into the table.