Hi there - looking for some help sorting linked records by ascending date in an automation script. Tried using sorts in the query, but no luck so far. Appreciate it!
Solved
Sorting linked records in automation script for summary
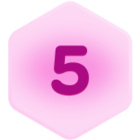
Best answer by Autumn_Grassel
I just did this yesterday! In my context, I had a table of People (our customers) that have registered for multiple events, and each registration record is linked.
I wanted the registration records to be linked and ordered by the date of the event people registered for. In my code, the 'Registrations' field is the linked record field that contains the records I wanted to sort.
The general steps I followed were:
- Get the list of linked records from the cell value of the linked record field
- For each linked record in the list, add an attribute for the date (or other field) that you want to sort by
- Sort the list of linked records by the attribute just added
- For each linked record, delete the attribute that was added
- Update the original record to store the now sorted list of records in the linked record field.
Below is some sample code for a Run Script action in an automation. It requires that a record Id is included as an input variable.
// get recordId that triggered automation from
// input variables
let recordId = input.config()['recordId'];
// Change this name to use a different table
let peopleTable = base.getTable("Customers");
let registrationsTable = base.getTable('Registrations');
// Prompt the user to pick a record
// If this script is run from a button field, this will use the button's record instead.
let record = await peopleTable.selectRecordAsync(recordId)
if (record) {
output.text(`You selected this record: ${record.name}`);
// get cell with linked records
let linkedRegistrations = record.getCellValue('Registrations');
if (linkedRegistrations) {
for (let registration of linkedRegistrations) {
// get the full linked record info
let registrationRecord = await registrationsTable.selectRecordAsync(registration.id);
registration['date'] = registrationRecord.getCellValue('start date')
}
// sorts list by date from earliest date to latest date
linkedRegistrations.sort((a, b) => (a.date > b.date) ? 1 : -1)
// remove the added date attributes after sorting
for (let registration of linkedRegistrations){
if (registration.date) {
delete registration.date;
}
}
// output.inspect(linkedRegistrations)
await peopleTable.updateRecordAsync(
record.id,
{
Registrations: linkedRegistrations
}
)
}
}
Reply
Enter your E-mail address. We'll send you an e-mail with instructions to reset your password.