Kim_Zhou wrote:
I see, how would I go about grabbing those interaction dates and comparing to new interaction date in the second option you listed? I would prefer to not make more fields unless I have to!
I did try the first option you listed and came up with this:
let inputConfig = input.config();
let table = base.getTable("Companies");
let records = inputConfig.triggerRecords
let interactionDate = inputConfig.interactionDate
let lastDate = inputConfig.lastDate
for (let record of records) {
await table.updateRecordAsync(record, {
if(lastDate < interactionDate){
"Date of Last Interaction": interactionDate
}, else: {
"Date of Last Interaction": lastDate
}
})
}
But have run into some errors since lastDate and interactionDates are strings. Also, I get “no overload matches this call” when I hover over the if statement.
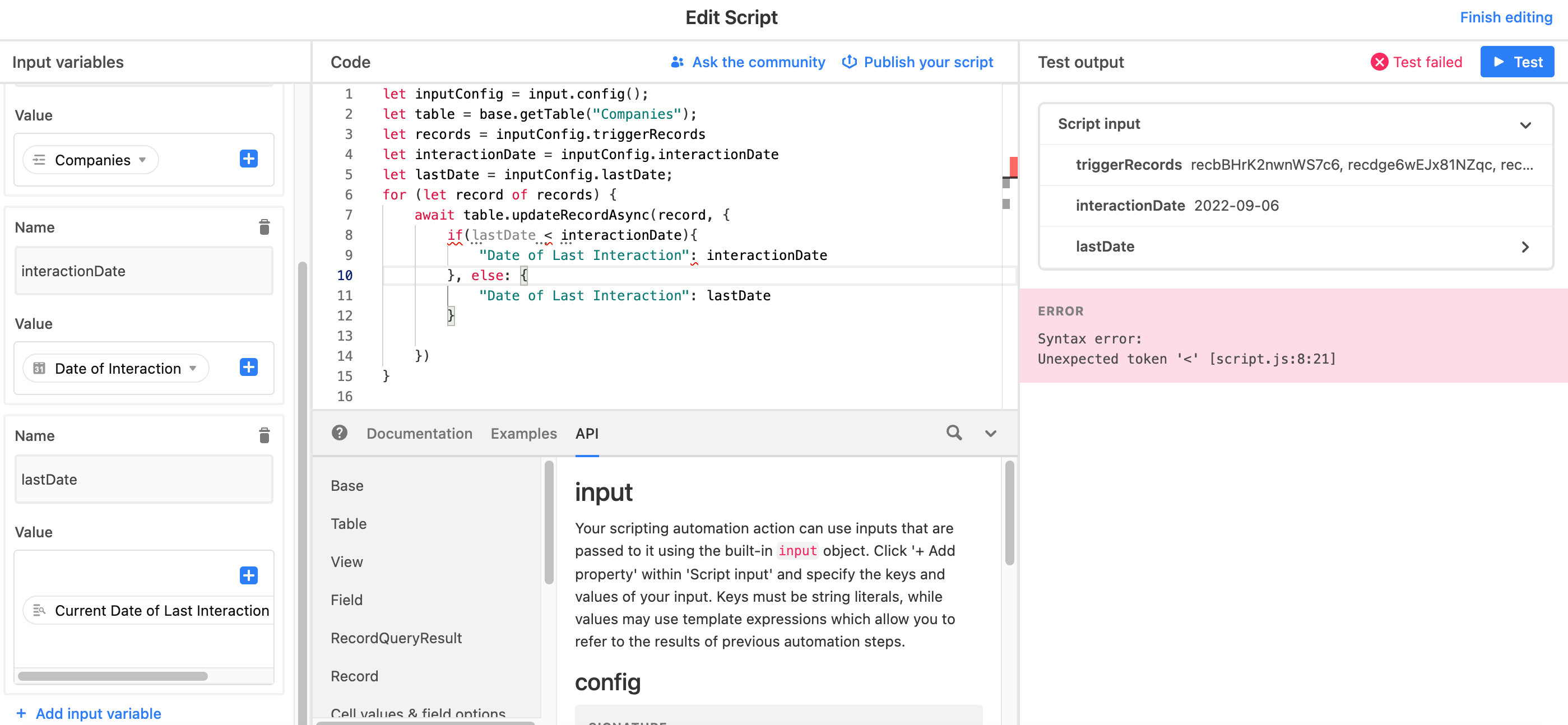
You’d need to do a selectRecordsAsync
to grab the triggerRecords, and for each of them, do a getCellValue
and compare them against interaction date
Here’s a base that does that; you should be able to see the code in the automation
Hmm, I don’t think you can use an IF
like that in an object?
I assume lastDate
is all the Date of Last Interaction
values of the linked company records? If so, you’re going to need to use some sort of i++ loop to refer to the data in your triggerRecords
and lastDate
values I think
e.g.
(I haven’t tested the below chunk, but conceptually I think it’s right)
let interactionDate = new Date(inputConfig.interactionDate)
for (let i = 0; i < triggerRecords; i++){
let companyInteractionDate = new Date(lastDate[i])
if (companyInteractionDate.getTime() < interactionDate.getTime()){
await table.updateRecordAsync(triggerRecords[i], {
"Date of Last Interaction": interactionDate
})
}
else... etc etc